Shopify + Zapier: How to itemize Order Line Item Properties
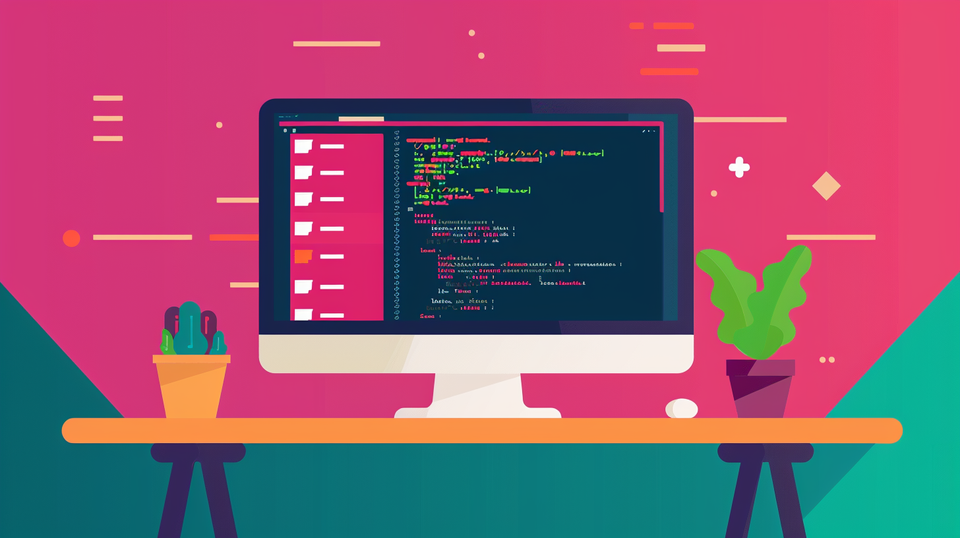
Table of contents
Zapier does not handle Shopify's Order Line Item Properties well. It gives us a total of three options:
Line Item Property Names
Line Item Property Values
Line Item Properties
All three are text (string) fields. Sadly, trying to merge the Names (keys) and Values objects doesn't work well as Zapier's tools and utilities do NOT handle data as well as we need it to (specifically: how to separate out a comma-delimited text where the value has a comma in it).
We tried using Zapier's utility
steps with many varieties and options and sadly, none of it did the job of getting us a nice key-value array or object back.
Solution
Thus, let's just do it ourselves. You can use the following JavaScript code in a Zapier Code step (of course with JS selected as the code language).
In the inputs of the code step, call your variable whatever you want, we called it rawData
and give it the value of the 'raw' properties (which shows up as empty when selecting it):
let properties = {};
let items = inputData.rawData.split(/\n\s*\n/);
items.forEach(function(item){
let temp = item.split(/\n/);
let name = temp[0].split(':')[1];
let value = temp[1].split(':')[1];
properties[name] = value;
});
output = properties;
Code explanation
let properties = {};
First, we declare an empty dictionary array. This will let us use named keys, rather than a regular array, which would only use an index.
let items = inputData.rawData.split(/\n\s*\n/);
Now we get the line item properties (called by inputData.rawData
) and split it by empty lines.
For whatever reason Zapier renders Shopify's line item properties as a text (string) with "Name: <name of field here>" and "Value: <users answer here>" in the following format:
Name: My First Example Field Title Here
Value: asjkdfasjkdhfaksjldfh
Name: Second field title or label here
Value: the second field's answe here and so forth
Thus, we split it by empty line so we can isolate each question-answer pair.
items.forEach(function(item){
let temp = item.split(/\n/);
let name = temp[0].split(':')[1];
let value = temp[1].split(':')[1];
properties[name] = value;
});
We then loop through this returned array (called items
) and split it by new line. Since the name and values are split by … a new line.
From there, we use that temporary value and further split the 2 value (first and second) array by colon :
. Specifically: we split it so that we can ignore the repeated text of 'Name: ' and 'Value: ' and thus getting us the actual values we want for each.
Then we insert (create) into the properties
dict array by key.
And of course, we then simply return that dict. Now you have an easily addressable object in Zapier you can use in further steps.